Syntax For Dev C++
It follows the same syntax as regular Markdown code blocks, with ways to tell the highlighter what language to use for the code block. The language will be detected automatically, if possible. Or you can specify it on the first line with 3 colons and the language name. C: If and Else Statements. So we've learnt how to collect basic data from the user, but wouldn't it be useful if we could do different things depending on what the user typed in? Well this happens to be a very core concept of computer programming, and we can do exactly as previously described with these things called 'if' statements. If-else Statement (C); 2 minutes to read +2; In this article. Controls conditional branching. Statements in the if-block are executed only if the if-expression evaluates to a non-zero value (or TRUE). If the value of expression is nonzero, statement1 and any other statements in the block are executed and the else-block, if present, is skipped. If the value of expression is zero. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type, specify the name of the array followed by square brackets and specify the number of elements it should store.
- C Programming Tutorial
- C Programming useful Resources
- Selected Reading
You have seen the basic structure of a C program, so it will be easy to understand other basic building blocks of the C programming language.
Tokens in C
A C program consists of various tokens and a token is either a keyword, an identifier, a constant, a string literal, or a symbol. For example, the following C statement consists of five tokens −
The individual tokens are −
Semicolons
In a C program, the semicolon is a statement terminator. That is, each individual statement must be ended with a semicolon. It indicates the end of one logical entity.
Given below are two different statements −

Comments
Comments are like helping text in your C program and they are ignored by the compiler. They start with /* and terminate with the characters */ as shown below −
You cannot have comments within comments and they do not occur within a string or character literals.
Identifiers
A C identifier is a name used to identify a variable, function, or any other user-defined item. An identifier starts with a letter A to Z, a to z, or an underscore '_' followed by zero or more letters, underscores, and digits (0 to 9).
C does not allow punctuation characters such as @, $, and % within identifiers. C is a case-sensitive programming language. Thus, Manpower and manpower are two different identifiers in C. Here are some examples of acceptable identifiers −
Keywords
The following list shows the reserved words in C. These reserved words may not be used as constants or variables or any other identifier names.
auto | else | long | switch |
break | enum | register | typedef |
case | extern | return | union |
char | float | short | unsigned |
const | for | signed | void |
continue | goto | sizeof | volatile |
default | if | static | while |
do | int | struct | _Packed |
double |
Whitespace in C
A line containing only whitespace, possibly with a comment, is known as a blank line, and a C compiler totally ignores it.
Whitespace is the term used in C to describe blanks, tabs, newline characters and comments. Whitespace separates one part of a statement from another and enables the compiler to identify where one element in a statement, such as int, ends and the next element begins. Therefore, in the following statement −
there must be at least one whitespace character (usually a space) between int and age for the compiler to be able to distinguish them. On the other hand, in the following statement −
no whitespace characters are necessary between fruit and =, or between = and apples, although you are free to include some if you wish to increase readability.
C++ is a popular programming language because it’s powerful, fast, easy to use, standardized, and more. Whether you are new to C++ programming or an advanced user, check out the following information on C++ mistakes, header files, and syntax. All information pertains to Windows, Mac, and Linux computers.
The 10 Most Common C++ Mistakes
Although many C++ programmers take measures to prevent bugs, mistakes still slip through. This list of the ten most common mistakes while writing C++ code can help both new and veteran programmers:
Sep 13, 2018 In this free video, Russ from Studio One Expert explains all the new features found in Studio One 4.1, these include;. Support for ATOM Production and Performance Pad Controller. New.
You forgot to declare the variable.
You used the wrong uppercase and lowercase letters; for example, you typed Main when you meant main.
You used one equal sign (=) when you were supposed to use two (), either in an if statement or in a for loop.
You forgot #include <iostream> or using namespace std;.
You dropped the laptop in the swimming pool.
You forgot to call new and just started using the pointer anyway.
You forgot the word public: in your classes so everything turned up private.
You let the dog eat the remote.
You forgot to type the parentheses when calling a function that takes no parameters.
You forgot a semicolon, probably at the end of a class declaration.
The Usual C++ Header Files
In C++, a header file holds forward declarations of identifiers. Here are some of the most common C++ header files that you’ll be using, along with their correct spellings. These aren’t by any means all of them, but they are the most common:
Include <string> if you’re going to be using the string class.
Include <iostream> when you want to use cout and cin.
Include <fstream> when you want to read or write files.
Include <iomanip> if you want advanced manipulator usage in your streams.
Include <stdlib.h> for general operations, including system(“PAUSE”).
C++ Syntax that You May Have Forgotten
Dev C++ For Windows 10
Remembering a bunch of C++ syntax can make you “loopy.” The following samples show the syntax of some of the more easily forgotten C++ situations: a for loop, a while loop, and a switch statement; a class and the code for a member function; a base class and a derived class; a function, function pointer type, and pointer to the function; and a class template and then a class based on the template.
Here’s a for loop:
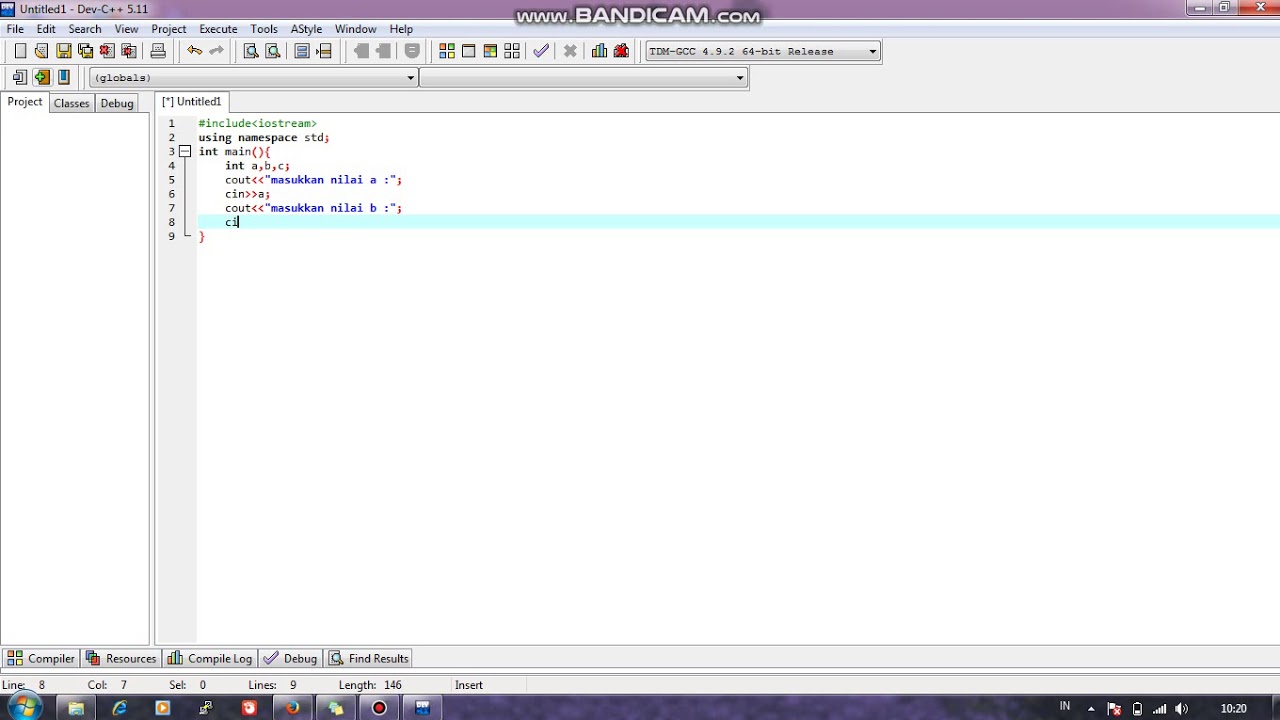
Here’s a while loop that counts from 10 down to 1:
And here’s a switch statement:
Here’s a class and the code for a member function:
Here’s a base class and a derived class:
Here’s a function, a function pointer type, and a pointer to the function:
Syntax For Decode In Sql
And here’s a class template and then a class based on the template: